The Option Storage is one of the core components of the Automation Platform architecture, and it is accessible as a system interface. This article describes the typical design pattern used to read and write those option values.
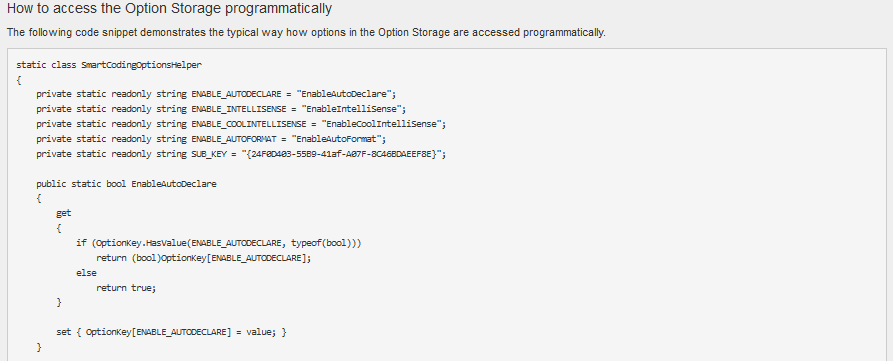
The structure of the Option Storage
The structure of the option storage is quite similar to the Windows registry. It consists of an arbitrary hierarchy of keys, and the leafs of the tree are named and typed values. The root of the key hierarchy describes the scope of the options below. The following roots are defined:
Root key | Storage location | Load time | Save time |
Project | Within the CODESYS project file | After the project has been loaded | Each time the project is saved |
Machine | %PROGRAMDATA%\CoDeSys\CoDeSys.opt (*) | During CODESYS startup | During CODESYS shutdown |
MachineProject | projectpath\projectnamewithoutext-AllUsers.opt-AllUsers.opt | After the project has been loaded | Each time the project is saved |
User | %APPDATA%\CoDeSys\CoDeSys.opt (*) | During CODESYS startup (**) | During CODESYS shutdown (***) |
UserProject | projectpath\projectnamewithoutext-username-domainname.opt | After the project has been loaded | Each time the project is saved |
(*) The substring CoDeSys might be overwritten by an OEM customization hook.
(**) When a user starts CoDeSys for the first time, the initial set of options are created by copying the file at %PROGRAMDATA%\CoDeSys\CoDeSysUser.opt. If that path does not yet exist, they are created by copying the file at installroot\Settings\CoDeSysUser.opt (unless overwritten by OEM customization hooks).
(***) Each time user options are written, the default user options at %PROGRAMDATA%\CoDeSys\CoDeSysUser.opt are modified as well. Effectively, a new user sees the same options as the last user that worked with CODESYS.
How to access the Option Storage programmatically
The following code snippet demonstrates the typical way how options in the Option Storage are accessed programmatically.
static class SmartCodingOptionsHelper
{
private static readonly string ENABLE_AUTODECLARE = "EnableAutoDeclare";
private static readonly string ENABLE_INTELLISENSE = "EnableIntelliSense";
private static readonly string ENABLE_COOLINTELLISENSE = "EnableCoolIntelliSense";
private static readonly string ENABLE_AUTOFORMAT = "EnableAutoFormat";
private static readonly string SUB_KEY = "{24F0D403-55B9-41af-A07F-8C46BDAEEF8E}";
public static bool EnableAutoDeclare
{
get
{
if (OptionKey.HasValue(ENABLE_AUTODECLARE, typeof(bool)))
return (bool)OptionKey[ENABLE_AUTODECLARE];
else
return true;
}
set { OptionKey[ENABLE_AUTODECLARE] = value; }
}
public static bool EnableIntelliSense
{
get
{
if (OptionKey.HasValue(ENABLE_INTELLISENSE, typeof(bool)))
return (bool)OptionKey[ENABLE_INTELLISENSE];
else
return true;
}
set { OptionKey[ENABLE_INTELLISENSE] = value; }
}
public static bool EnableCoolIntelliSense
{
get
{
if (OptionKey.HasValue(ENABLE_COOLINTELLISENSE, typeof(bool)))
return (bool)OptionKey[ENABLE_COOLINTELLISENSE];
else
return false;
}
set { OptionKey[ENABLE_COOLINTELLISENSE] = value; }
}
public static bool EnableAutoFormat
{
get
{
if (OptionKey.HasValue(ENABLE_AUTOFORMAT, typeof(bool)))
return (bool)OptionKey[ENABLE_AUTOFORMAT];
else
return true;
}
set { OptionKey[ENABLE_AUTOFORMAT] = value; }
}
private static IOptionKey OptionKey
{
get { return SystemInstances.OptionStorage.GetRootKey(OptionRoot.User).CreateSubKey(SUB_KEY); }
}
}
Using that code pattern, you code can access the options in a convenient and type-safe way by simply calling something like SmartCodingOptionsHelper.EnableAutoFormat. Of course you can use other datatypes than bool as well. All datatypes that can be used with the CoDeSys standard serialization mechanism are possible in the option storage as well.
Make the settings persistent
As long as you are modifying Machine, MachineProject, User, or UserProject options, there is nothing special to do in order to persist the data. The Automation Platform architecture ensures that the data is stored appropriately according to the table above.
Things are a little bit different for Project options. This is the only root where the options are not stored in a kind of sidecar file, but in the project itself, namely in the workspace object (which can be edited by clicking the "Project Settings" either in the menu or in the navigator). Unfortunately, the mechanisms of getting a writable copy of that workspace object and committing it to the Object Manager again is not automatically done, so you'll have to wrap your code like that:
IMetaObject mo = SystemInstances.ObjectMgr.GetObjectToModify(nProjectHandle, GUID_WORKSPACEOBJECT);
// modify the option storage here...
MemoryStream stream = new MemoryStream();
SystemInstances.OptionStorage.Save(OptionRoot.Project, stream);
stream.Close();
IWorkspaceObject workspaceObject = (IWorkspaceObject)mo.Object;
workspaceObject.OptionData = stream.ToArray();
SystemInstances.ObjectMgr.SetObject(mo, true, null);
private static readonly Guid GUID_WORKSPACEOBJECT = new Guid("{6470A90F-B7CB-43ac-9AE5-94B2338B4573}");
Please note that you might get an exception while getting the modifiable copy (the object might be under source control, write-restricted for the current user, or in use by any other editor).
Reacting to changes
Your plugin should not rely on the options being modified exclusively through the option editors or your own code. Other code (like the SVN operations "Switch" and "Update") may change the project options. The IOptionStorage interface provides appropriate events (OptionCreated, OptionChanged and OptionDeleted) you can use to keep informed when the options change.
created by Kevin Ketterle